Elevate Your VBA Skills: Variables As Dynamic Form Components
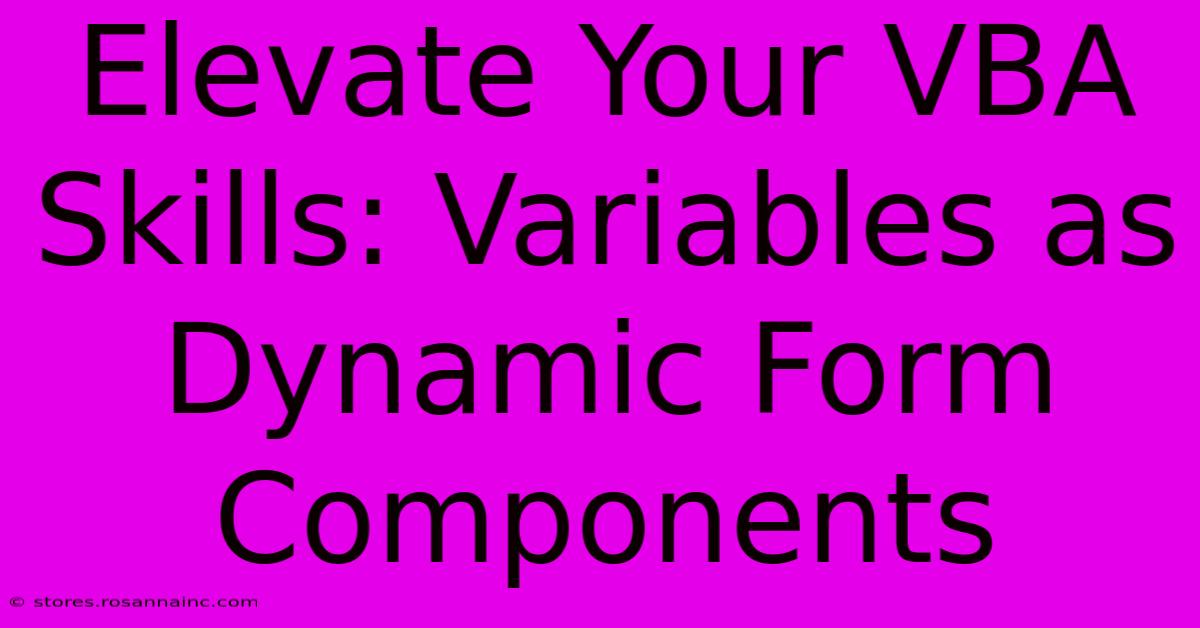
Table of Contents
Elevate Your VBA Skills: Variables as Dynamic Form Components
Are you tired of static, inflexible forms in your VBA projects? Do you dream of forms that adapt and change based on user input or data? Then it's time to explore the power of using variables as dynamic form components in VBA! This technique opens up a world of possibilities, allowing you to create truly responsive and user-friendly applications. This comprehensive guide will show you how to leverage this powerful technique to take your VBA skills to the next level.
Understanding the Power of Dynamic Forms
Traditional VBA forms often involve a fixed set of controls – text boxes, labels, buttons – placed directly onto the form's design surface. While functional, this approach lacks flexibility. Imagine needing to add or remove controls based on user actions or data retrieved from a database. Manually managing this would be cumbersome and error-prone.
Dynamic forms, however, offer a solution. By using variables to create and manipulate form controls at runtime, you gain unparalleled control over your user interface. This means you can:
- Create controls on-the-fly: Add text boxes, buttons, or any other control type based on specific conditions.
- Modify existing controls: Change the properties of controls (like caption, size, or visibility) dynamically.
- Remove controls: Cleanly remove controls that are no longer needed.
- Build adaptive interfaces: Create forms that intelligently adjust their layout and functionality based on user interactions or data changes.
Building Your First Dynamic Form Component
Let's start with a simple example: creating a dynamic text box using a variable.
Sub CreateDynamicTextBox()
Dim txtBox As TextBox
' Create a new text box
Set txtBox = Controls.Add("Forms.TextBox.1", "DynamicTextBox")
' Position the text box
txtBox.Left = 100
txtBox.Top = 100
' Set other properties (optional)
txtBox.Width = 200
txtBox.Height = 20
txtBox.Text = "This is a dynamic text box!"
End Sub
This code snippet first declares a variable txtBox
of type TextBox
. Then, using the Controls.Add
method, it creates a new text box, assigning it to the txtBox
variable. Finally, it sets the text box's position and other properties. This new text box is now fully functional and part of your form, all created at runtime!
Expanding Functionality: Dynamic Label Creation
Let's build upon this by dynamically creating a label to accompany our text box:
Sub CreateDynamicTextBoxAndLabel()
Dim txtBox As TextBox
Dim lbl As Label
' Create the text box (as before)
Set txtBox = Controls.Add("Forms.TextBox.1", "DynamicTextBox")
txtBox.Left = 100
txtBox.Top = 100
txtBox.Width = 200
txtBox.Height = 20
' Create the label
Set lbl = Controls.Add("Forms.Label.1", "DynamicLabel")
lbl.Left = 100
lbl.Top = 80
lbl.Caption = "Enter your text here:"
End Sub
Now you've created a label and a text box dynamically! The label provides context for the text box, demonstrating how easily you can create interconnected components.
Advanced Techniques: Arrays and Loops for Complex Forms
For more complex forms, you can leverage arrays and loops to create multiple controls dynamically. Imagine a scenario where you need to display data from a database. Instead of manually adding text boxes for each data point, you can use loops to create them efficiently.
Sub CreateDynamicTextboxesFromArray(data() As String)
Dim i As Long
Dim txtBox As TextBox
For i = 0 To UBound(data)
Set txtBox = Controls.Add("Forms.TextBox.1", "DynamicTextBox" & i)
txtBox.Left = 100
txtBox.Top = 100 + i * 25 ' Adjust vertical position
txtBox.Text = data(i)
Next i
End Sub
This example takes an array of strings (data
) and dynamically creates a text box for each element in the array. The loop automatically adjusts the vertical position of each text box, ensuring they don't overlap.
Error Handling and Best Practices
Remember to always include error handling in your code. This will prevent unexpected crashes and improve the robustness of your application. Always check if the Controls.Add
method was successful and handle any potential errors gracefully.
Using variables to create dynamic form components is a powerful technique that adds significant flexibility and adaptability to your VBA applications. By mastering these techniques, you'll be able to create more sophisticated and user-friendly applications that truly respond to user needs and data changes. Experiment with these examples, explore the different properties you can control, and push the boundaries of what's possible with your VBA projects!
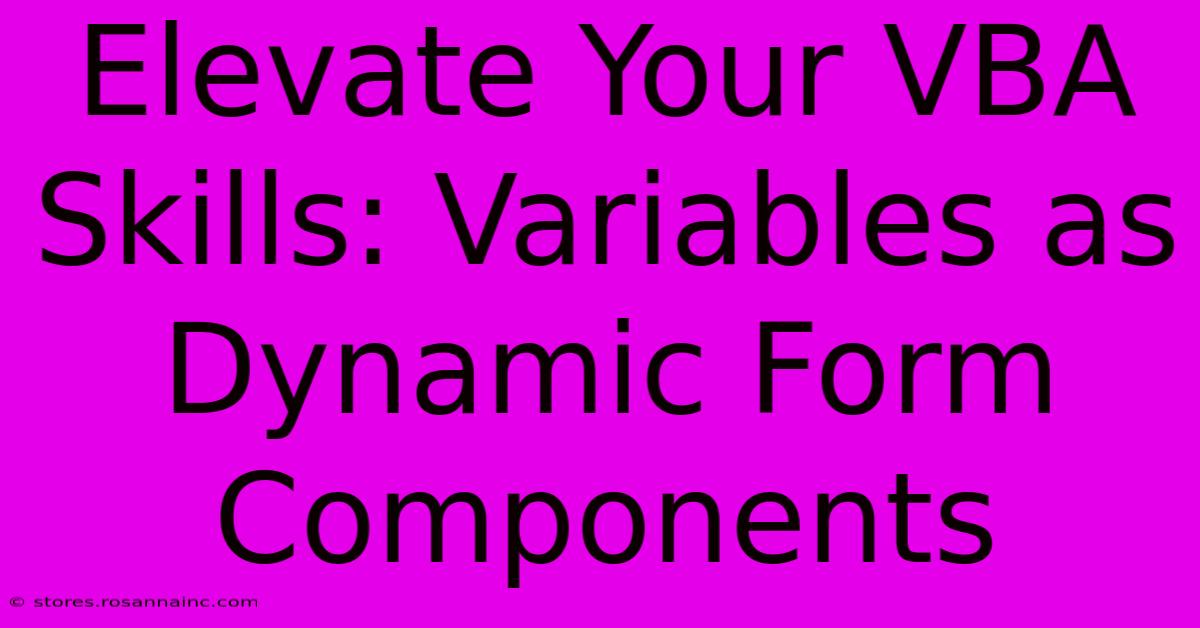
Thank you for visiting our website wich cover about Elevate Your VBA Skills: Variables As Dynamic Form Components. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Behold The Most Horrifying Mascots Lurking On College Campuses
Feb 06, 2025
-
Prepare For Mind Boggling The Team That Will Leave You Scratching Your Head
Feb 06, 2025
-
Amber Haze
Feb 06, 2025
-
From Scratch To Pro The Ultimate Guide To 3 D Modeling Education
Feb 06, 2025
-
The Ultimate Guide To Using The Porsche Font Unlock Its Power
Feb 06, 2025